Are you ready to embark on a journey into the heart of Linux command line mastery? Welcome to the world of Bash scripting – a powerful and versatile tool for automating tasks, managing systems, and unleashing the full potential of your Linux environment. In this comprehensive guide, we’ll take you from the basics of Bash scripting to advanced techniques, equipping you with the knowledge and skills to become a proficient Bash scripter. Whether you’re a system administrator, developer, or power user, Bash scripting is an indispensable skill for maximizing productivity and efficiency on the command line.
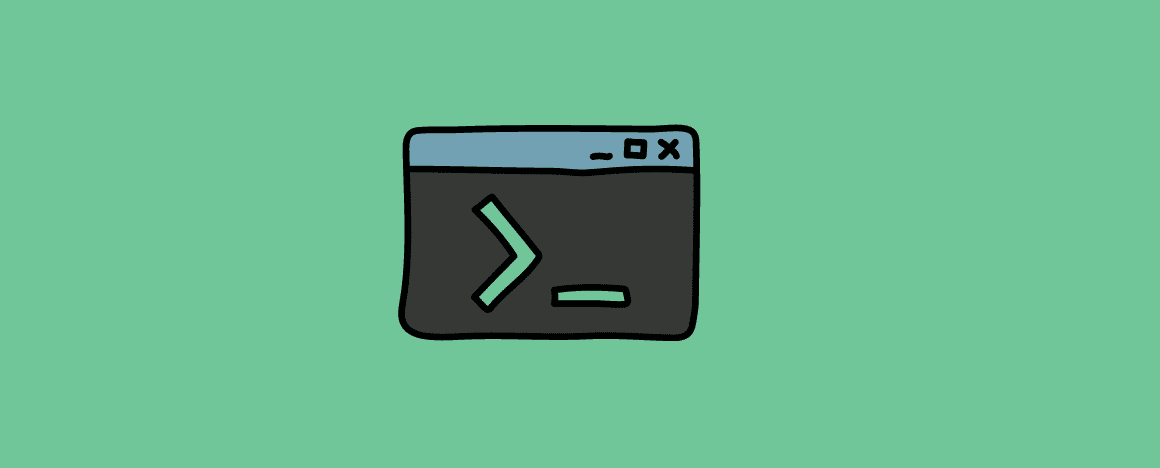
Why Bash Scripting?
Bash, short for “Bourne Again Shell,” is the default shell for most Linux distributions. It provides a robust and flexible environment for executing commands and writing scripts. By mastering Bash scripting, you can automate repetitive tasks, simplify complex operations, and streamline your workflow. Whether you’re managing servers, developing applications, or performing system maintenance, Bash scripting empowers you to accomplish tasks with speed and efficiency.
Advantages of Bash Script
Bash script is a powerful and flexible tool for automating system administration tasks, managing system resources, and performing other common tasks in Unix systems /Linux.
Some of the benefits of shell scripts are: Automation: Shell scripts allow you to automate repetitive tasks and processes, saving time and reducing the risk of possible errors generated during manual execution.
Portability: The Shell script can run on a variety of platforms and operating systems, including Unix, Linux, macOS, and even Windows through the use of emulators or virtual machines.
Flexibility: Shell scripts are highly customizable and can be easily modified to meet specific requirements. They can also be combined with other programming languages or utilities to create more powerful scripts.
Accessibility: Shell scripts are easy to write and do not require any special tools or software. They can be edited with any text editor, and most operating systems have a built-in shell interpreter.
Integration: Shell scripts can be integrated with other tools and applications, such as databases, web servers, and cloud services, allowing for system management tasks to be performed on more complex systems and automation.
Debugging: Shell scripts are easy to debug, and most shells have built-in debugging and error reporting tools that can help quickly identify and fix problems.
An overview of Command Line Interface and Bash Shell
The terms “shell” and “bash” are often used interchangeably, but they have subtle differences.
A “shell” is a program that offers a command-line interface for interacting with an operating system. Bash (Bourne-Again SHell) stands as one of the most prevalent Unix/Linux shells and is the default in many Linux distributions.
When the shell operates as root (an administrative user), the prompt changes to #, presenting a superuser shell prompt like this:
[root@host ~]#
Although Bash is a type of shell, there exist other shells, such as Korn shell (ksh), C shell (csh), and Z shell (zsh). Each shell possesses its syntax and features, but they all serve the common purpose of furnishing a command-line interface for OS interaction.
You can identify your shell type using the ps command:
ps
In essence, while “shell” is a broad term referring to any program offering a command-line interface, “Bash” is a specific type of shell widely utilized in Unix/Linux systems.
Note: Throughout this tutorial, we’ll be utilizing the “bash” shell.
Getting Started with Bash
Before diving into Bash scripting, it’s essential to have a solid foundation in using the command line interface. Familiarize yourself with basic commands for navigating the filesystem, managing files and directories, and interacting with the shell. Once you’re comfortable with the basics, you’re ready to begin your journey into Bash scripting.
Basic Syntax and Structure
Remarks regarding bash scripting
Comments in bash scripting begin with a #. This indicates that the interpreter will disregard any line that starts with a # as a comment.
Adding comments to the code is a good practice because they aid in code documentation and make the code easier for others to understand.
Here are some instances of remarks:
#The interpreter will disregard both of these lines;
#This is an example comment.
Bash scripts are plain text files containing a series of commands and instructions to be executed by the Bash shell. They typically start with a shebang (‘#!/bin/bash’) followed by the script’s code. Comments can be added using the ‘#’ symbol to annotate the script for clarity and documentation purposes. Let’s explore the basic syntax and structure of Bash scripts:
#!/bin/bash
# This is a simple Bash script
echo "Hello, world!"
In this example, we’ve created a basic Bash script that prints “Hello, world!” to the terminal when executed. The ‘echo’ command is used to display text, and the shebang specifies the path to the Bash interpreter.
Variables and Data Manipulation
Variables in Bash are used to store data and can be referenced or manipulated throughout the script. Variable names are case-sensitive and can contain letters, numbers, and underscores. To assign a value to a variable, use the syntax ‘variable_name=value’. Let’s explore how to work with variables in Bash:
name="John"
echo "Hello, $name!"
In this example, we’ve created a variable named ‘name’ and assigned it the value “John”. We then use the ‘echo’ command to print a greeting message using the value stored in the ‘name’ variable.
Bash also supports various data manipulation techniques, such as string concatenation, substring extraction, and arithmetic operations, allowing you to manipulate data efficiently within your scripts.
Variable Naming Conventions
The following are the variable name conventions used in Bash scripting:
- Initial letters or underscores (_) are appropriate for variable names.
- Underscores (_), numerals, and characters are all permitted in variable names.
- The case of variable names matters.
- Special characters or spaces should not appear in variable names.
- Make sure the variable’s names are clear and represent its intended use.
- Don’t name variables with reserved terms like if, then, else, fi, and so forth.
For example:
num
list
my_Var
myInfo
Basic Bash Commands
- echo: Display text to the terminal.
- cd: Change the current directory to a specified location.
- ls: List the contents of the current directory.
- mkdir: Create a new directory.
- touch: Create a new file.
- rm: Remove a file or directory.
- cp: Copy a file or directory.
- mv: Move or rename a file or directory.
- cat: Concatenate and display the contents of a file.
- grep: Search for a pattern in a file.
- chmod: Modify the permissions of a file or directory.
- sudo: Execute a command with administrative privileges.
- df: Show the amount of available disk space.
- history: Display a list of previously executed commands.
- ps: Show information about running processes.
Gathering Input
Reading User Input and Storing in a Variable
To capture user input, we employ the read command. Below is an example script:
#!/bin/bash
echo "Today is $(date)"
echo -e "\nEnter the path to the directory:"
read the_path
echo -e "\nFiles and folders in your provided path:"
ls "$the_path"
Reading from a File
This snippet reads each line from a file named input.txt and outputs it to the terminal using a while loop:
while read line
do
echo "$line"
done < input.txt
Command Line Arguments
In a bash script or function, positional parameters denote the arguments passed. $1 represents the first argument, $2 the second, and so forth.
Below is an example script greeting.sh that takes a name as a command-line argument and produces a personalized greeting:
#!/bin/bash
echo "Hello, $1!"
To execute this script with the name "Alan" as the argument:
./greeting.sh Alan
This will result in the output:
Hello, Alan!
Displaying Output
Printing to the Terminal
The ‘echo’ command is used to display text on the terminal. For example:
echo “Hello, World!”
When executed, this command prints the text “Hello, World!” to the terminal.
Writing to a File
You can also direct the output of ‘echo’ to a file instead of the terminal. This is done using the ‘>’ operator, which redirects the output to a file. For instance:
echo "This is Message." > output.txt
Here, the text “This is some text.” will be written to a file named output.txt. If the file already exists, its previous content will be overwritten.
Appending to a File
If you want to add text to an existing file without erasing its current contents, you can use the ‘>>’ operator. For example:
echo "More Message." >> output.txt
This command appends the text “More text.” to the end of the file output.txt, preserving its previous content.
Redirecting Output
Besides ‘echo’, you can redirect the output of any command to a file. For instance, the ‘ls’ command lists the files in the current directory. You can redirect this output to a file like so:
ls > files.txt
This command lists the files in the current directory and writes the output to a file named files.txt. Similarly, you can redirect the output of various other commands to files for further processing or documentation.
If-Else Statements
If-else statements allow you to perform actions based on conditions. They are structured as follows:
age=18
if [ $age -ge 18 ]; then
echo "You are an adult."
else
echo "You are a minor."
fi
In this example, we use the ‘-ge’ operator to check if the value of the ‘age’ variable is greater than or equal to 18. If the condition is true, the script prints “You are an adult.” Otherwise, it prints “You are a minor.”
For Loops
For loops allow you to iterate over a sequence of values and perform actions for each value. They are structured as follows:
for i in {1..5}; do
echo "Number: $i"
done
In this example, the loop iterates over the values 1 through 5 and prints each value to the terminal.
While Loops
While loops execute commands repeatedly as long as a specified condition is true. They are structured as follows:
count=0
while [ $count -lt 5 ]; do
echo "Count: $count"
((count++))
done
In this example, the loop increments the ‘count’ variable by 1 each iteration and prints its value to the terminal until the ‘count’ reaches 5.
Functions and Modularization
Functions allow you to modularize your scripts by grouping related commands together. They can take arguments and return values, providing a way to encapsulate logic and promote code reusability. Let’s explore how to define and use functions in Bash:
greet() {
echo "Hello, $1!"
}
greet "Alice"
In this example, we define a function named ‘greet’ that takes a single argument (‘$1’) and prints a greeting message using the value of the argument.
Advanced Topics in Bash Scripting
Once you’ve mastered the basics of Bash scripting, you can explore more advanced topics to further enhance your skills and capabilities. These topics include file manipulation, process management, error handling, debugging techniques, and more. Let’s briefly explore some of these advanced topics:
File Manipulation
Bash provides a variety of commands and tools for working with files and directories, such as ‘cp’, ‘mv’, ‘rm’, ‘mkdir’, ‘rmdir’, and ‘touch’. You can use these commands to create, copy, move, rename, delete, and manipulate files and directories as needed.
Process Management
Bash allows you to manage processes and execute commands in the background using features like job control, process substitution, and process redirection. You can use commands like ‘ps’, ‘kill’, ‘bg’, ‘fg’, and ‘nohup’ to monitor, control, and manipulate processes on your system.
Error Handling
Error handling is an essential aspect of Bash scripting, ensuring that your scripts gracefully handle unexpected errors and failures. You can use constructs like ‘trap’, ‘exit’, and ‘error handling’ to detect, handle, and recover from errors in your scripts.
Debugging Techniques
Debugging Bash scripts can be challenging, but Bash provides several tools and techniques to help you identify and resolve issues efficiently. You can use options like ‘-x’ for debugging mode, ‘set -e’ for exit on error, and ‘set -u’ for treating unset variables as errors to debug your scripts effectively.
Integrating External Tools and Utilities
Bash scripting allows you to leverage a vast ecosystem of external tools and utilities to extend the capabilities of your scripts. You can invoke external commands, call other scripts, and integrate with other programming languages like Python, Perl, and Ruby to accomplish complex tasks and solve specific problems.
Customizing Your Scripts
One of the strengths of Bash scripting is its flexibility and customizability. You can tailor your scripts to suit specific requirements and scenarios by using command-line arguments, environment variables, configuration files, and user input. By making your scripts configurable and adaptable, you can ensure they meet the needs of your users and environments.
Best Practices and Tips
As you continue to develop your Bash scripting skills, keep the following best practices and tips in mind:
Use descriptive variable names: Choose meaningful names for your variables to improve readability and maintainability of your scripts. This makes it easier for you and others to understand the purpose and function of each variable.
Comment your code: Add comments to explain the logic, purpose, and functionality of your code. This helps other developers (and your future self) understand the script’s behavior and intent.
Handle errors gracefully: Implement error handling mechanisms to detect and handle errors and failures gracefully. This includes validating user input, checking for the existence of files or directories, and handling unexpected errors with appropriate error messages and actions.
Test your scripts: Thoroughly test your scripts under various conditions and scenarios to ensure they behave as expected. Test for edge cases, invalid inputs, and unexpected conditions to identify and address potential issues before deploying your scripts in production environments.
Use version control: Utilize version control systems like Git to track changes to your scripts, collaborate with other developers, and manage revisions and updates effectively. Version control helps you maintain a history of changes and revert to previous versions if needed.
Document your scripts: Document your scripts with clear and concise explanations of their purpose, usage, and dependencies. Include information on how to install, configure, and run the script, as well as any required dependencies or external tools.
Stay up-to-date: Keep abreast of new features, updates, and best practices in Bash scripting and Linux system administration. Follow online communities, forums, and resources to learn from others, ask questions, and share your knowledge and experiences.
Wrapping Up
Congratulations! You’ve completed your journey into the world of Bash scripting. Armed with the knowledge and skills you’ve acquired, you’re now equipped to automate tasks, manage systems, and unleash the full potential of your Linux environment through the power of Bash scripting. Whether you’re a seasoned sysadmin, a budding developer, or an enthusiastic power user, Bash scripting is a valuable skill that will serve you well in your Linux journey.
As you continue to explore and experiment with Bash scripting, remember to keep learning, practicing, and pushing the boundaries of what you can achieve. Embrace challenges, seek out new opportunities, and never stop expanding your horizons. With dedication, perseverance, and a dash of creativity, you’ll continue to refine your Bash scripting skills and unlock new possibilities on the command line.
So go forth, write scripts, solve problems, and make the most of your Linux experience. The world is your oyster, and with Bash scripting as your trusty companion, there’s no limit to what you can accomplish. Happy scripting!